Contents
Control Statements
If- Expression
Decision Making in programming is similar to decision-making in real life. In programming too, a certain block of code needs to be executed when some condition is fulfilled. A programming language uses control statements to control the flow of execution of a program based on certain conditions. If the condition is true then it enters into the conditional block and executes the instructions.
There are different types of if-else expressions in Kotlin:
- if expression
- if-else expression
- if-else-if ladder expression
- nested if expression
If- Expression : Kotlin’s if
can be used both as a statement and as an expression. When used as an expression, it returns a value.
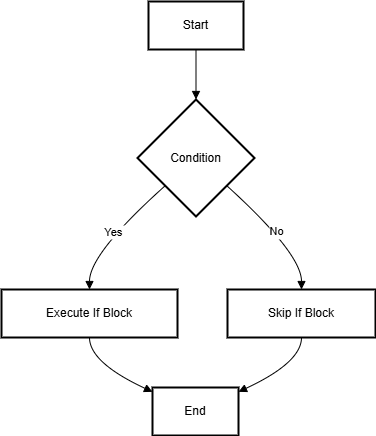
Syntax:
if(condition) {
// code to run if condition is true
}
Example:
fun main(args: Array) {
var a = 3
if(a > 0){
print("Yes,number is positive")
}
}
Output:
Yes, number is positive
if-else-if ladder Expression: Kotlin’s if-else-if ladder can be used both as a statement and as an expression. When used as an expression, it returns a value.
Syntax:
if(Firstcondition) {
// code to run if condition is true
}
else if(Secondcondition) {
// code to run if condition is true
}
else{
}
Example:
import java.util.Scanner
fun main(args: Array) {
// create an object for scanner class
val reader = Scanner(System.`in`)
print("Enter any number: ")
// read the next Integer value
var num = reader.nextInt()
var result = if ( num > 0){
"$num is positive number"
}
else if( num < 0){
"$num is negative number"
}
else{
"$num is equal to zero"
}
println(result)
}
Output:
Enter any number: 12
12 is positive number
Enter any number: -11
-11 is negative number
Enter any number: 0
0 is zero
If-Else Expression: Kotlin’s if-else
can be used both as a statement and as an expression. When used as an expression, it returns a value.
Syntax:
if(condition) {
// code to run if condition is true
}
else {
// code to run if condition is false
}
Example:
if(condition) {
// code to run if condition is true
}
else {
// code to run if condition is false
}
Output:
fun main(args: Array) {
var a = 5
var b = 10
if(a > b){
print("Number 5 is larger than 10")
}
else{
println("Number 10 is larger than 5")
}
}
nested if expression: Kotlin’s if-else-if ladder can be used both as a statement and as an expression. When used as an expression, it returns a value.
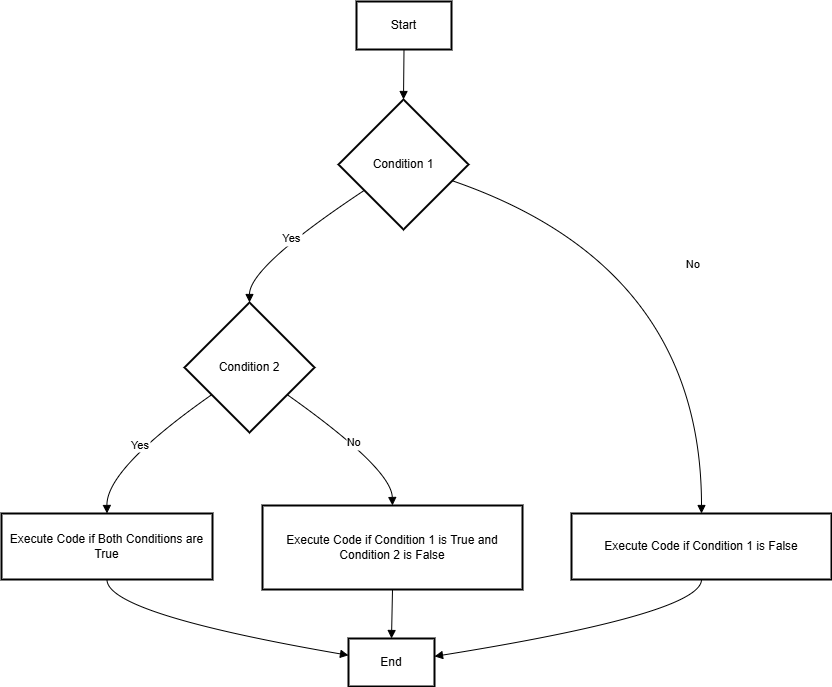
Syntax:
if(condition1){
// code 1
if(condition2){
// code2
}
}
Example:
import java.util.Scanner
fun main(args: Array) {
// create an object for scanner class
val reader = Scanner(System.`in`)
print("Enter three numbers: ")
var num1 = reader.nextInt()
var num2 = reader.nextInt()
var num3 = reader.nextInt()
var max = if ( num1 > num2) {
if (num1 > num3) {
"$num1 is the largest number"
}
else {
"$num3 is the largest number"
}
}
else if( num2 > num3){
"$num2 is the largest number"
}
else{
"$num3 is the largest number"
}
println(max)
}
Output:
Enter three numbers: 123 231 321
321 is the largest number
While Loop
The while
loop executes a block of code as long as the condition is true.
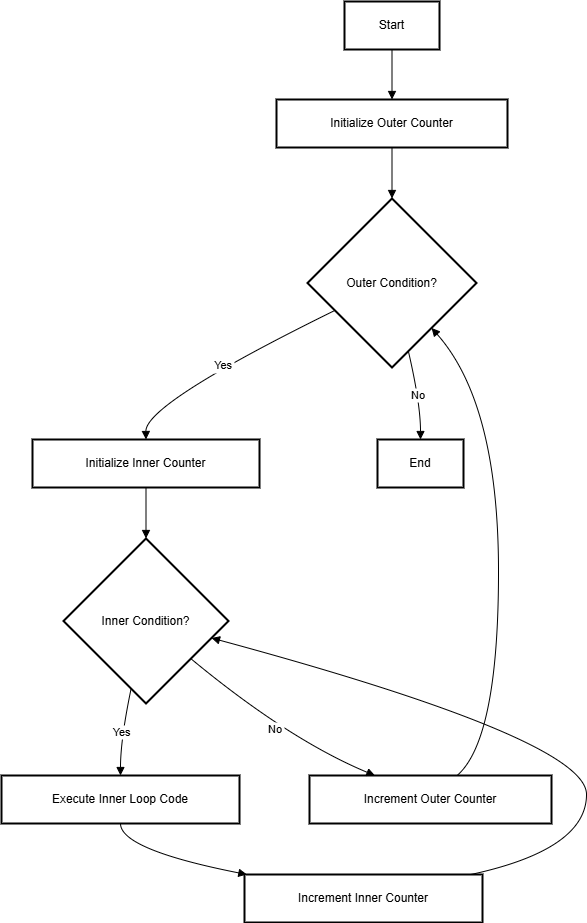
Syntax:
fun main(args: Array) {
var number = 1
while(number <= 10) {
println(number)
number++;
}
}
Example:
var count = 5
while (count > 0) {
println("Count: $count")
count--
}
Output:
Count: 5
Count: 4
Count: 3
Count: 2
Count: 1
Do-While Loop
The do-while
loop executes the block of code at least once before checking the condition.
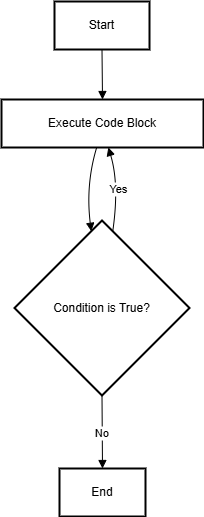
Syntax:
while(condition) {
// code to run
}
Example:
var count = 5
do {
println("Count: $count")
count--
} while (count > 0)
Output:
Count: 5
Count: 4
Count: 3
Count: 2
Count: 1
For Loop
Kotlin’s for
loop is used to iterate over ranges, arrays, or other iterable objects.
Syntax:
for(item in collection) {
// code to execute
}
Example:
// Iterating over a range
for (i in 1..5) {
println(i)
}
// Iterating over an array
val items = arrayOf("apple", "banana", "cherry")
for (item in items) {
println(item)
}
// Iterating with an index
for ((index, value) in items.withIndex()) {
println("Item at $index is $value")
}
Output:
1
2
3
4
5
apple
banana
cherry
Item at 0 is apple
Item at 1 is banana
Item at 2 is cherry
When Expression
The when
expression in Kotlin is used as a replacement for the switch statement and can be used both as a statement and as an expression.
Syntax:
fun main (args : Array) {
print("Enter the name of heavenly body: ")
var name= readLine()!!.toString()
when(name) {
"Sun" -> print("Sun is a Star")
"Moon" -> print("Moon is a Satellite")
"Earth" -> print("Earth is a planet")
else -> print("I don't know anything about it")
}
}
Example:
val x = 3
when (x) {
1 -> println("x is 1")
2 -> println("x is 2")
3, 4 -> println("x is 3 or 4")
in 5..10 -> println("x is in the range 5 to 10")
!in 1..10 -> println("x is outside the range 1 to 10")
else -> println("x is none of the above")
}
// When as an expression
val result = when (x) {
1 -> "One"
2 -> "Two"
else -> "Unknown"
}
println(result)
Output:
x is 3 or 4
Unknown
Unlabelled Break
The break
statement terminates the nearest enclosing loop.
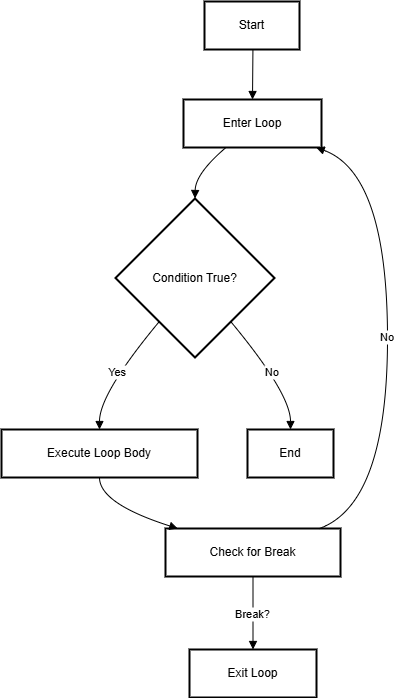
Syntax:
while(test expression) {
// code to run
if(break condition) {
break
}
// another code to run
}
Example:
fun main(args: Array) {
var sum = 0
var i = 1
while(i <= Int.MAX_VALUE) {
sum += i
i++
if(i == 11) {
break
}
}
print("The sum of integers from 1 to 10: $sum")
}
Output:
The sum of integers from 1 to 10: 55
labelled Break
The continue
statement skips the current iteration of the nearest enclosing loop. With labels, you can control which loop to continue.
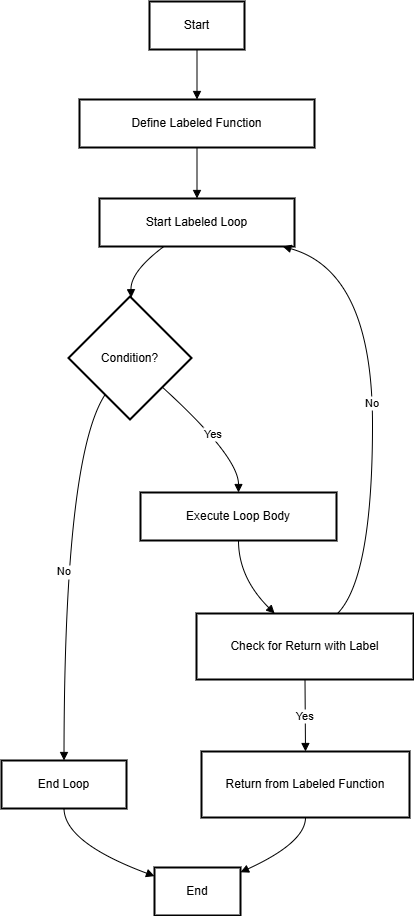
Syntax:
outer@ while(firstcondition) {
// code
inner@ while(secondcondition) {
//code
if(condition for continue) {
continue@outer
}
}
}
Example:
outer@ for (i in 1..5) {
for (j in 1..5) {
if (i == 3 && j == 2) {
println("Breaking outer loop at i=$i, j=$j")
break@outer // Breaks the outer loop
}
println("i=$i, j=$j")
}
}
Output:
i=1, j=1
i=1, j=2
i=1, j=3
i=1, j=4
i=1, j=5
i=2, j=1
i=2, j=2
i=2, j=3
i=2, j=4
i=2, j=5
i=3, j=1
Breaking outer loop at i=3, j=2