Contents
Flow Control in java
Decision Making in Java (if, if-else, switch, break, continue, jump).
Decision-making in programming is like making decisions in real life. When coding, we often need to execute certain blocks of code only if specific conditions are met.
Java provides several control statements to control the flow of a program based on conditions. These statements direct the flow of execution, allowing it to branch and continue based on the program’s state.
Java’s Selection Statements:
if
if-else
nested-if
if-else-if
switch-case
jump
(break, continue, return)
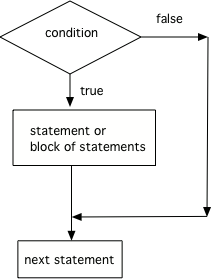
1. if Statement:
The simplest decision-making statement. It is used to decide whether a certain block of code will be executed or not, depending on whether the condition is true
.
Syntax:
if(condition) {
// Statements to execute if the condition is true
}
Here, the condition
evaluates to either true
or false
. If true
, the block of statements inside the if
block is executed.
If we do not use curly braces ({}
), only the first statement after the if
will be considered part of the if
block.
Example:
class IfDemo {
public static void main(String args[]) {
int i = 10;
if (i < 15)
System.out.println("Inside If block"); // Part of if block
System.out.println("10 is less than 15"); // Outside of if block
System.out.println("I am not in if block");
}
}
Output:
Inside If block
10 is less than 15
I am not in if block
2. if-else Statement:
The if
statement alone can execute code when a condition is true
. But what if we want to execute something else when the condition is false
? This is where the else
statement comes in.
Syntax:
if (condition) {
// Executes if condition is true
} else {
// Executes if condition is false
}
Example:
class IfElseDemo {
public static void main(String args[]) {
int i = 10;
if (i < 15)
System.out.println("i is smaller than 15");
else
System.out.println("i is greater than 15");
}
}
Output:
i is smaller than 15
3. nested-if Statement:
A nested-if
is an if
statement inside another if
. This allows you to perform more complex decision-making scenarios.
Syntax:
if (condition1) {
if (condition2) {
// Executes when both conditions are true
}
}
Example:
class NestedIfDemo {
public static void main(String args[]) {
int i = 10;
if (i == 10 || i < 15) {
if (i < 15)
System.out.println("i is smaller than 15");
if (i < 12)
System.out.println("i is smaller than 12 too");
} else {
System.out.println("i is greater than 15");
}
}
}
Output:
i is smaller than 15
i is smaller than 12 too
4. if-else-if Ladder:
The if-else-if
ladder allows you to test multiple conditions. The conditions are evaluated from top to bottom, and as soon as one of them is true
, its corresponding block is executed, and the rest are skipped.
Syntax:
if (condition1) {
// Executes if condition1 is true
} else if (condition2) {
// Executes if condition2 is true
} else {
// Executes if none of the above conditions are true
}
Example:
class IfElseIfDemo {
public static void main(String args[]) {
int i = 20;
if (i == 10)
System.out.println("i is 10");
else if (i == 15)
System.out.println("i is 15");
else if (i == 20)
System.out.println("i is 20");
else
System.out.println("i is not present");
}
}
Output:
i is 20
5. switch-case Statement:
The switch
statement allows you to branch execution based on the value of an expression. It’s like having multiple if-else
blocks for a single expression.
Syntax:
switch (expression) {
case value1:
// Code to be executed if expression equals value1
break;
case value2:
// Code to be executed if expression equals value2
break;
// Add more cases as needed
default:
// Code to be executed if none of the cases match
}
Example:
class SwitchDemo {
public static void main(String[] args) {
int num = 20;
switch (num) {
case 5:
System.out.println("It is 5");
break;
case 10:
System.out.println("It is 10");
break;
case 15:
System.out.println("It is 15");
break;
case 20:
System.out.println("It is 20");
break;
default:
System.out.println("Not present");
}
}
}
Output:
It is 20
6. Jump Statements:
Java has three main jump statements:
- break: Used to exit a loop or a switch statement.
- continue: Skips the remaining code in the current iteration of a loop and starts the next iteration.
- return: Exits from the current method and passes control back to the caller.
Example of continue
:
class ContinueDemo {
public static void main(String args[]) {
for (int i = 0; i < 10; i++) {
if (i % 2 == 0)
continue;
System.out.print(i + " ");
}
}
}
Output:
1 3 5 7 9
If Statements with Examples
Decision Making in Java allows you to execute a specific block of code based on certain conditions. It’s a key part of controlling the flow of a program, similar to how we make decisions in real life.
Java’s if
Statement:
The if
statement is the simplest form of decision-making. It allows you to execute a block of code if a specific condition evaluates to true.
Syntax:
if (condition) {
// Statements to execute if the condition is true
}
How It Works:
1. Control enters the if block.
2. The condition is evaluated.
- If the condition is true, the block of code within the if statement is executed.
- If the condition is false, the control moves to the next part of the program.
Example:
class IfDemo {
public static void main(String args[]) {
int i = 10;
if (i < 15)
System.out.println("10 is less than 15");
System.out.println("Outside if-block");
}
}
Output:
10 is less than 15
Outside if-block
Java if-else
Statement
Decision-making in Java allows for the execution of certain blocks of code based on specific conditions. The if
statement tells us that if a condition is true, it will execute a block of statements; otherwise, it won’t. By combining if
and else
, you can handle both outcomes—what happens when the condition is true and when it’s false.
Understanding if-else
in Java:
The if-else
statement is used to control the flow of your program by executing code based on whether a condition is true or false.
Syntax of if-else
Statement:
if (condition) {
// Executes this block if the condition is true
} else {
// Executes this block if the condition is false
}
Example Program:
// Java program to demonstrate if-else statement
class IfElseDemo {
public static void main(String args[]) {
int i = 20;
if (i < 15) {
System.out.println("i is smaller than 15");
} else {
System.out.println("i is greater than 15");
}
System.out.println("Outside if-else block");
}
}
Output:
i is greater than 15
Outside if-else block
Java if-else-if
Ladder
Decision-making in Java allows us to write condition-based statements that execute particular blocks of code depending on specific conditions. The if-else-if
ladder is used to handle multiple conditions by checking each one sequentially. As soon as a condition evaluates to true
, its corresponding block is executed, and the rest of the ladder is ignored. If none of the conditions are true, the final else
block is executed.
Syntax:
if (condition) {
// Executes if condition 1 is true
} else if (condition) {
// Executes if condition 2 is true
}
.
.
else {
// Executes if no conditions are true
}
Working of if-else-if
Ladder:
1. Control enters the if block.
2. The first condition is evaluated:If true, the associated block is executed, and the rest of the ladder is skipped.
If false, the flow moves to the next condition.
3. This process repeats for each condition in the ladder.
4. If none of the conditions are met, the else block is executed.
Example :
// Java program to demonstrate if-else-if ladder
class IfElseIfLadderDemo {
public static void main(String[] args) {
// Initializing expression
int i = 20;
// Condition 1
if (i == 10) {
System.out.println("i is 10");
}
// Condition 2
else if (i == 15) {
System.out.println("i is 15");
}
// Condition 3
else if (i == 20) {
System.out.println("i is 20");
}
// Default case
else {
System.out.println("i is not present");
}
System.out.println("Outside if-else-if ladder");
}
}
Output:
i is 20
Outside if-else-if ladder
Loops in Java
Looping is an essential feature in programming that allows a set of instructions to be executed repeatedly as long as a specified condition evaluates to true. In Java, loops are implemented in three different ways, each with a unique syntax and method of checking conditions.
while Loop
Looping is an essential feature in programming that allows a set of instructions to be executed repeatedly as long as a specified condition evaluates to true. In Java, loops are implemented in three different ways, each with a unique syntax and method of checking conditions.
while (boolean condition) {
// Loop body
}
Example:
public class WhileLoopExample {
public static void main(String[] args) {
int i = 0;
while (i <= 10) {
System.out.println(i);
i++;
}
}
}
Output:
0
1
2
3
4
5
6
7
8
9
10
Working of while
loop:
- The loop starts by checking the boolean condition.
- If the condition is
true
, the loop body is executed. - After each execution, the condition is rechecked before the next iteration.
- The loop terminates when the condition evaluates to
false
.
This is an entry control loop, meaning the condition is checked before the loop body is executed.
Java do-while loop with Examples
Loops are a fundamental concept in programming, used when we need to execute a block of code repeatedly. The do-while
loop is an example of an Exit Control Loop in Java, meaning the loop body will always execute at least once, as the condition is checked after the loop body has been executed, unlike in for
and while
loops where the condition is checked beforehand.
Syntax of do-while
Loop:
do {
// Loop body
update_expression;
} while (test_expression);
Output:
Print statement
For-each loop in Java
The for-each loop in Java, introduced in Java 5, is a simplified way to traverse arrays and collections. It provides a cleaner and more readable syntax when you need to access each element of an array or a collection like ArrayList
.
Key Features of the For-Each Loop:
- It begins with the keyword
for
, similar to a regular for-loop. - Instead of initializing and maintaining a loop counter, you declare a variable (of the same type as the array elements) that iterates through each element.
- The loop body uses this variable directly, without needing to reference an array index.
The for-each loop is particularly useful for iterating through arrays or classes from the Java Collection Framework, such as ArrayList
.
Syntax:
for (type var : array) {
// Statements using var
}
Example: Simple Array Traversal using For-Each Loop
public class Easy {
public static void main(String[] args) {
// Array declaration
int[] ar = {10, 50, 60, 80, 90};
// For-each loop to iterate through array
for (int element : ar) {
System.out.print(element + " ");
}
}
}
Output:
10 50 60 80 90
Equivalent Syntax with Regular For Loop:
for (int i = 0; i < ar.length; i++) {
int element = ar[i];
// Statements using element
}
Example: Finding the Highest Score using For-Each Loop
// Java program to illustrate for-each loop
public class ForEachExample {
public static void main(String[] args) {
int[] marks = {125, 132, 95, 116, 110};
int highestMarks = maximum(marks);
System.out.println("The highest score is " + highestMarks);
}
public static int maximum(int[] numbers) {
int maxSoFar = numbers[0];
// For-each loop to find the maximum value
for (int num : numbers) {
if (num > maxSoFar) {
maxSoFar = num;
}
}
return maxSoFar;
}
}
Output:
The highest score is 132
Limitations of the For-Each Loop
1. Modifying Array Elements: For-each loops don’t allow modification of array elements. Changing the loop variable does not affect the array:
for (int num : marks) {
num = num * 2; // Only changes num, not the actual array element
}
2. No Access to Index: You can’t get the index of the current element using a for-each loop:
for (int num : numbers) {
if (num == target) {
return ???; // No index available
}
}
3. Only Forward Iteration: For-each loops can only iterate forward, making it impossible to iterate in reverse order:
for (int i = numbers.length - 1; i >= 0; i--) {
System.out.println(numbers[i]); // Cannot be done using for-each
}
4. Handling Multiple Decision Statements: For-each loops struggle to process multiple decision-making conditions:
for (int i = 0; i < numbers.length; i++) {
if (numbers[i] == arr[i]) {
// Hard to convert this to a for-each loop
}
}
Break Statement in Java
The break statement in Java is a control statement used to terminate the loop prematurely. Once the break
statement is encountered, the loop terminates immediately, and the control moves to the next statement following the loop.
Syntax:
break;
When to Use the Break Statement:
1. When the exact number of iterations is not known in advance.
2. When you want to exit the loop based on some condition during iteration.
Use Cases for Break:
1. Terminating a sequence in a switch statement.
2. Exiting a loop.
3. Serving as a structured alternative to goto.
Example: Break in a Switch Statement
// Example of using break statement in switch
public class SwitchBreakExample {
public static void main(String[] args) {
// Assigning n an integer value
int n = 1;
// Passing n to switch
// The case matching n will execute and terminate due to break
switch (n) {
case 1:
System.out.println("Hello World");
break;
case 2:
System.out.println("Second Case");
break;
default:
System.out.println("Default Case");
}
}
}
Output:
Hello World
Using Break to Exit a Loop
With the break statement, you can force the loop to terminate immediately, skipping the remaining iterations. It’s commonly used when you want to stop the loop based on a specific condition.
Example: Breaking out of a Loop
// Java program to illustrate using break to exit a loop
public class BreakLoopDemo {
public static void main(String[] args) {
// The loop runs from 0 to 9
for (int i = 0; i < 10; i++) {
// Terminate loop when i equals 5
if (i == 5)
break;
System.out.println("i: " + i);
}
System.out.println("Loop complete.");
}
}
Output:
i: 0
i: 1
i: 2
i: 3
i: 4
Loop complete.
- Time Complexity: O(1)
- Auxiliary Space: O(1)
Using Break as a Form of Goto
Java doesn’t support the goto
statement because it allows jumping in an unstructured manner, leading to complex and unreadable code. Instead, Java uses labels to identify a block of code. You can use the break
statement to jump out of a labeled block.
Syntax:
label:
{
// Code block
statement1;
statement2;
// Break can be used to exit the block
break label;
}
Example: Break with a Label
// Java program to illustrate using break with label
public class BreakLabelDemo {
public static void main(String[] args) {
boolean condition = true;
// Label first
first: {
second: {
third: {
// Before break
System.out.println("Before the break statement");
// If condition is true, break out of the second block
if (condition)
break second;
System.out.println("This won't execute.");
}
System.out.println("This won't execute.");
}
// After exiting the second block
System.out.println("This is after second block.");
}
}
}
Output:
Before the break statement
This is after second block
return keyword in Java
In Java, the scope of a variable refers to the region in the code where the variable is accessible. Java has lexical (static) scoping, meaning the scope of a variable is determined at compile time and is not dependent on the function call stack. The scope rules in Java can be broadly classified into three categories based on where the variables are declared.
1. Member Variables (Class-Level Scope)
2. Local Variables (Method-Level Scope)
3. Block Variables (Loop or Block-Level Scope)
1. Member Variables (Class-Level Scope)
Member variables are declared inside a class but outside any method, constructor, or block. They can be accessed anywhere within the class and can have different access levels (e.g., public
, private
, protected
, or default). Access to member variables outside the class depends on the access modifier used.
Example:
public class Test {
// Member variables
int a; // Default access modifier
private String b; // Private member variable
char c; // Default access modifier
void method1() {
// Member variables can be accessed here
System.out.println(a);
System.out.println(b);
}
int method2() {
return a;
}
}
- Public: Accessible within the class, in subclasses, and outside the class.
- Protected: Accessible within the class and in subclasses but not outside the package.
- Default (no modifier): Accessible within the same package but not outside it.
- Private: Only accessible within the class.
2. Local Variables (Method-Level Scope)
Local variables are declared inside a method or constructor and are only accessible within that method. They must be initialized before use, and their lifetime is limited to the method’s execution. Once the method finishes, local variables are destroyed.
Example:
public class Test {
void method1() {
// Local variable
int x = 10;
System.out.println(x); // Accessible inside the method
}
public static void main(String[] args) {
Test t = new Test();
t.method1();
}
}
Local variables do not retain their values once the method completes, and they are recreated each time the method is invoked.
3. Block Variables (Loop or Block-Level Scope)
Variables declared inside a block (within curly braces {}
) are only accessible within that block. Once the block is exited, these variables are out of scope and cannot be accessed. This applies to variables declared inside loops or conditionals.
Example of Block-Level Scope:
public class Test {
public static void main(String[] args) {
{
int x = 10; // x is only accessible inside this block
System.out.println(x);
}
// Uncommenting the following line will cause an error
// System.out.println(x); // x is out of scope here
}
}
Loop Variables (Block Scope)
Variables declared inside a loop have scope limited to the loop. They cannot be accessed outside the loop.
Example:
class Test {
public static void main(String[] args) {
for (int x = 0; x < 4; x++) {
System.out.println(x); // x is accessible inside the loop
}
// Uncommenting the following line will result in an error
// System.out.println(x); // x is out of scope here
}
}
If you need to access a loop variable outside the loop, declare it before the loop:
class Test {
public static void main(String[] args) {
int x;
for (x = 0; x < 4; x++) {
System.out.println(x);
}
System.out.println(x); // x is accessible outside the loop
}
}
Output:
0
1
2
3
4
Loop Variable Scope with Overlapping Names
In Java, you cannot declare two variables with the same name within the same scope. However, in languages like C++, it’s possible to have the same variable name in nested scopes, which is not allowed in Java.
Incorrect Example in Java (compilation error):
class Test {
public static void main(String[] args) {
int a = 5;
for (int a = 0; a < 5; a++) { // Error: Variable 'a' is already defined
System.out.println(a);
}
}
}
Output:
Error: variable 'a' is already defined
Valid Example with Loop Variable Declaration After Loop
To avoid such errors, you can declare a variable outside the loop and use it after the loop finishes.
Example:
class Test {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
System.out.println(i); // Loop variable i
}
int i = 20; // Declare i after the loop
System.out.println(i); // Access new i outside the loop
}
}
Output:
1
2
3
4
5
6
7
8
9
10
20