Contents
Components in React
Components are the fundamental building blocks of a React application. They allow you to break down the UI into reusable and isolated pieces. There are two main types of components in React: function components and class components.
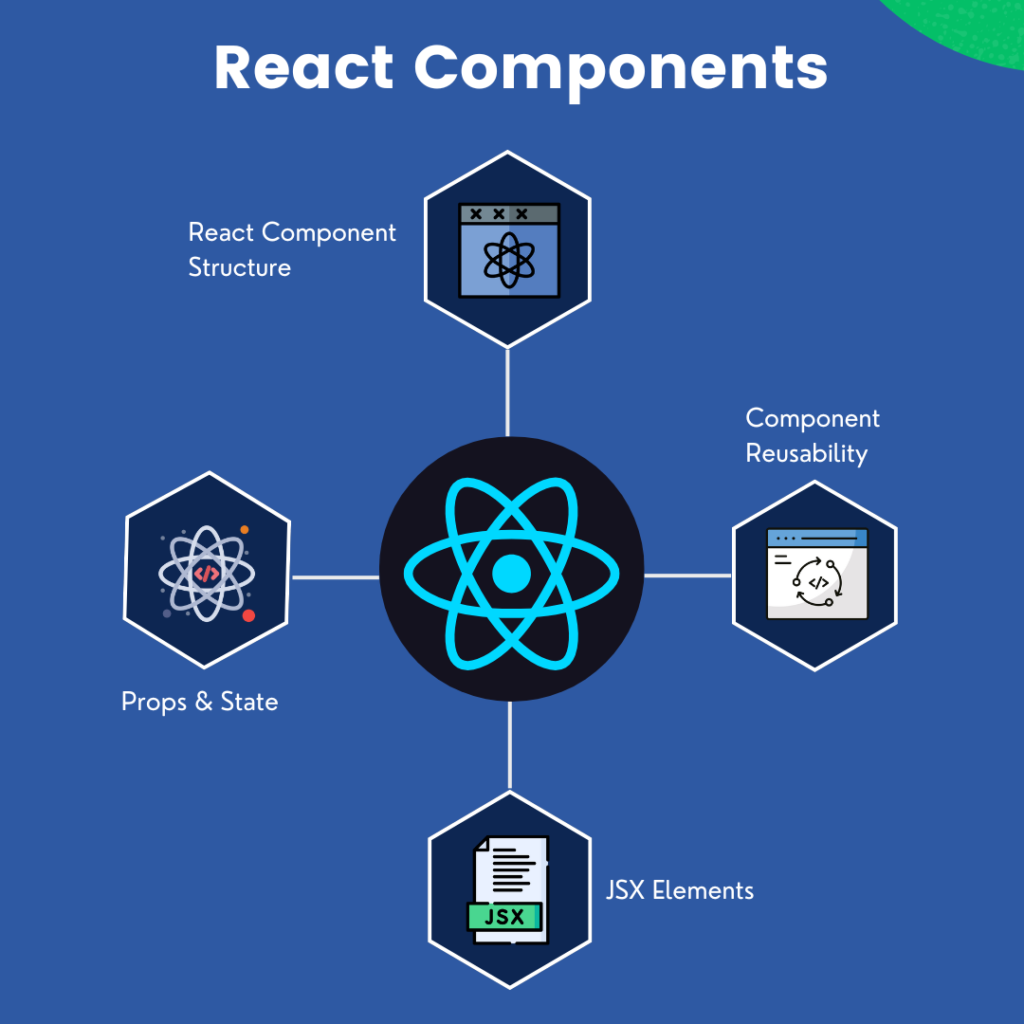
Function and Class Components
Function Components
Function components are the simpler of the two types. They are JavaScript functions that return JSX. Function components do not have their own state or lifecycle methods (prior to the introduction of hooks).
Here’s an example of a simple function component:
function Greeting(props) {
return Hello, {props.name}!
;
}
Class Components
Class components are more feature-rich than function components. They are ES6 classes that extend
React.Component
and must define arender
method that returns JSX.Here’s an example of a class component:
class Greeting extends React.Component {
render() {
return Hello, {this.props.name}!
;
}
}
Creating and Using Components
Creating Components
To create a component, simply define a function or a class that returns JSX. Each component should be placed in its own file, making it easier to manage and reuse.
For example, let’s create a
Greeting
component:- Function Component:
// Greeting.js
import React from 'react';
function Greeting(props) {
return Hello, {props.name}!
;
}
export default Greeting;
- Class Component:
// Greeting.js
import React from 'react';
class Greeting extends React.Component {
render() {
return Hello, {this.props.name}!
;
}
}
export default Greeting;
Using Components
To use a component, import it into another file and include it in the JSX of that file.
For example, using the
Greeting
component in anApp
component:
// App.js
import React from 'react';
import Greeting from './Greeting';
function App() {
return (
);
}
export default App;
Component Props and Default Props
Component Props
Props (short for properties) are a way to pass data from parent components to child components. They are read-only and cannot be modified by the child component.
For example, in the
Greeting
component above,props.name
is used to display the name passed from the parent component.Default Props
Default props allow you to set default values for props if they are not provided by the parent component.
Here’s how you can define default props for a function component:
Greeting.defaultProps = {
name: 'Guest'
};
And for a class component:
class Greeting extends React.Component {
static defaultProps = {
name: 'Guest'
};
render() {
return Hello, {this.props.name}!
;
}
}
Component State and setState Method
Component State
State is a way to manage data that can change over time within a component. State is private to the component and can be modified using the
setState
method.Here’s an example of a class component with state:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
return (
Count: {this.state.count}
);
}
}
export default Counter;
setState Method
The
setState
method is used to update the component’s state. It schedules an update to the component’s state object and tells React to re-render the component with the updated state.For example, in the
Counter
component above, clicking the button updates thecount
state and re-renders the component with the new count value.With the introduction of hooks, function components can also manage state using the
useState
hook:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
Count: {count}
);
}
export default Counter;
Conclusion
Components are the backbone of any React application. By understanding how to create and use function and class components, manage props and state, and leverage the setState
method, you can build powerful and dynamic user interfaces. As you continue to develop your React skills, you’ll find that components provide a flexible and reusable way to construct your application’s UI.