Contents
React Router
React Router is a powerful library for managing navigation and routing in React applications. It allows you to create single-page applications with multiple views and navigate between them seamlessly. In this section, we’ll cover setting up React Router, creating navigation with Link
and NavLink
, and defining and using route parameters.
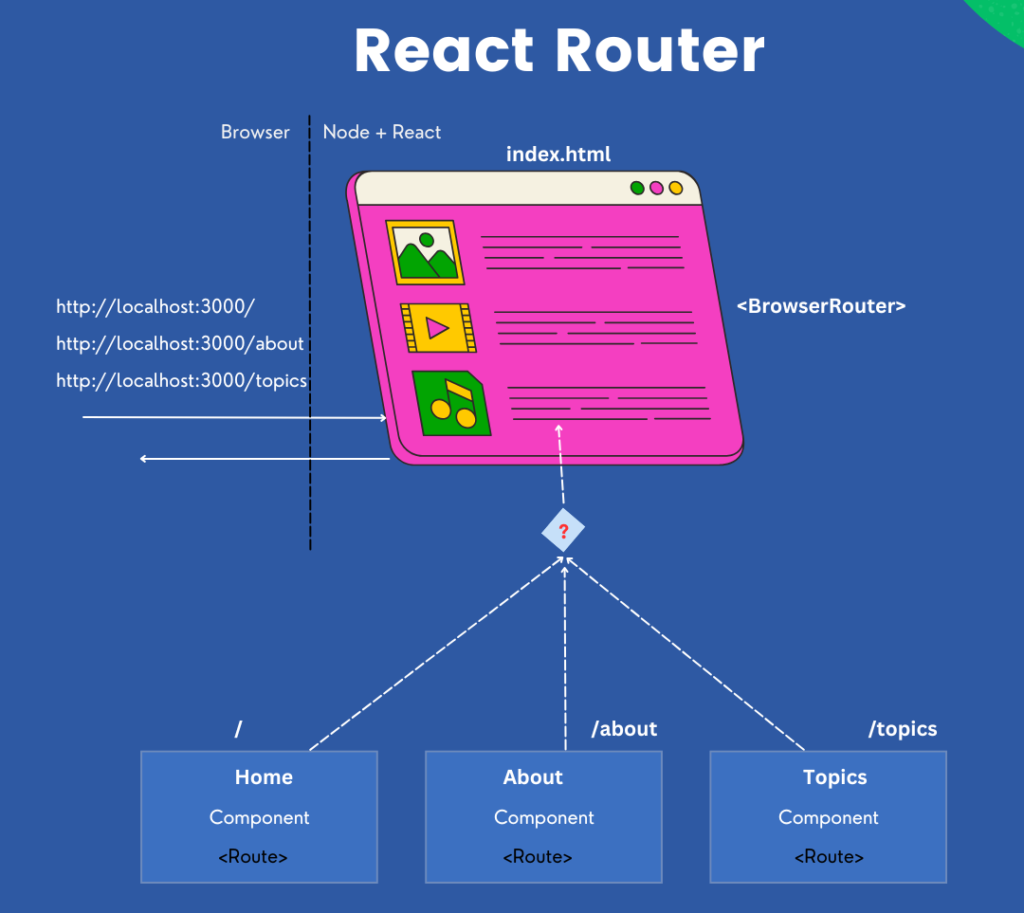
Setting Up React Router
To get started with React Router, you need to install the react-router-dom
package.
Step 1: Install React Router
npm install react-router-dom
Step 2: Set Up the Router
In your main application file (e.g., App.js
), set up the router by wrapping your application in BrowserRouter
and defining your routes using Route
.
Example:
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './Home';
import About from './About';
import Contact from './Contact';
function App() {
return (
);
}
export default App;
In this example:
BrowserRouter
is used to wrap the entire application, enabling routing capabilities.Route
is used to define individual routes, withpath
specifying the URL andcomponent
specifying the component to render.Switch
ensures that only one route is rendered at a time.
Creating Navigation with Link and NavLink
Link
and NavLink
components are used to create navigation links in your application.
Using Link
:
The Link
component is used to create navigation links that allow users to navigate to different routes.
Example:
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Switch, Link } from 'react-router-dom';
import Home from './Home';
import About from './About';
import Contact from './Contact';
function App() {
return (
);
}
export default App;
Using NavLink
:
The NavLink
component is similar to Link
but provides additional styling options for the active link.
Example:
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Switch, NavLink } from 'react-router-dom';
import Home from './Home';
import About from './About';
import Contact from './Contact';
function App() {
return (
);
}
export default App;
In this example, the activeClassName
prop is used to apply the active
class to the active link, allowing you to style it differently.
Defining and Using Route Parameters
Route parameters allow you to create dynamic routes that can accept input as part of the URL. These parameters can be accessed in the component using the match
object.
Example:
- Define the Route with Parameters:
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Switch, NavLink } from 'react-router-dom';
import Home from './Home';
import About from './About';
import Contact from './Contact';
import UserProfile from './UserProfile';
function App() {
return (
);
}
export default App;
2. Define the Route with Parameters:
// UserProfile.js
import React from 'react';
import { useParams } from 'react-router-dom';
function UserProfile() {
let { username } = useParams();
return (
User Profile
Username: {username}
);
}
export default UserProfile;
In this example:
- The route
/user/:username
defines a route parameterusername
. - The
useParams
hook is used within theUserProfile
component to access the route parameter and display it.
Conclusion
React Router is a powerful tool for managing navigation and routing in React applications. By setting up React Router, creating navigation with Link
and NavLink
, and defining and using route parameters, you can build dynamic and responsive single-page applications. These skills are essential for creating user-friendly and maintainable React applications.