Context API
The Context API in React is a powerful tool for managing global state and passing data through the component tree without the need for prop drilling. This means you can share data across multiple components at different nesting levels more easily.
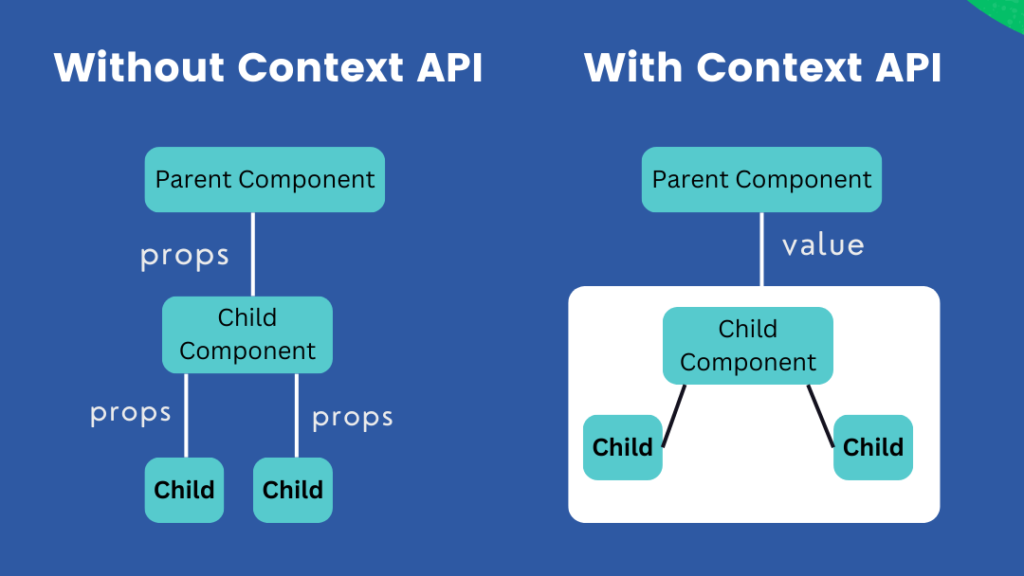
Introduction to the Context API
The Context API is designed to solve the problem of prop drilling, where you need to pass props through many intermediate components to reach the ones that need the data. It allows you to create a context, which holds the data you want to share, and then provide that context to components in the tree. These components can then consume the context and access the shared data directly.
Creating and Providing Context
To use the Context API, you first need to create a context and then provide it to your component tree.
Step 1: Create a Context
Create a context using React.createContext()
. This will return an object with Provider
and Consumer
components.
Example:
import React from 'react';
const ThemeContext = React.createContext('light');
export default ThemeContext;
In this example, ThemeContext
is created with a default value of 'light'
.
Step 2: Provide the Context
Wrap the components that need access to the context with the Provider
component. The Provider
component accepts a value
prop, which will be passed to the consuming components.
Example:
// App.js
import React, { useState } from 'react';
import ThemeContext from './ThemeContext';
import Toolbar from './Toolbar';
function App() {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
<Toolbar />
</ThemeContext.Provider>
);
}
export default App;
In this example, App
provides the theme
context to its child components. It also provides a toggleTheme
function to switch between themes.
Consuming Context in Components
There are two main ways to consume context in your components: using the Context.Consumer
component and using the useContext
hook.
Using Context.Consumer
The Context.Consumer
component allows you to consume the context in a class or function component. It expects a function as a child, which receives the context value.
Example:
// Toolbar.js
import React from 'react';
import ThemeContext from './ThemeContext';
import ThemedButton from './ThemedButton';
function Toolbar() {
return (
<div>
<ThemedButton />
</div>
);
}
export default Toolbar;
// ThemedButton.js
import React from 'react';
import ThemeContext from './ThemeContext';
function ThemedButton() {
return (
<ThemeContext.Consumer>
{({ theme, toggleTheme }) => (
<button
onClick={toggleTheme}
style={{
backgroundColor: theme === 'light' ? '#fff' : '#333',
color: theme === 'light' ? '#000' : '#fff',
}}
>
Toggle Theme
</button>
)}
</ThemeContext.Consumer>
);
}
export default ThemedButton;
In this example, ThemedButton
consumes the theme
and toggleTheme
values from the ThemeContext
.
Using useContext Hook
The useContext
hook provides a simpler and more modern way to consume context in function components.
Example:
// ThemedButton.js
import React, { useContext } from 'react';
import ThemeContext from './ThemeContext';
function ThemedButton() {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<button
onClick={toggleTheme}
style={{
backgroundColor: theme === 'light' ? '#fff' : '#333',
color: theme === 'light' ? '#000' : '#fff',
}}
>
Toggle Theme
</button>
);
}
export default ThemedButton;
In this example, ThemedButton
uses the useContext
hook to access the theme
and toggleTheme
values from the ThemeContext
.
Conclusion
The Context API is a powerful feature in React that simplifies state management and data sharing across your component tree. By creating and providing context, you can avoid prop drilling and make your components more modular and maintainable. Consuming context using the Context.Consumer
component or the useContext
hook allows you to access the shared data easily and efficiently.
Next, we’ll delve into React Hooks, which offer a way to use state and other React features in function components, making your code more concise and functional.