State Management with Redux
Redux is a popular state management library for JavaScript applications, commonly used with React. It helps manage the application state in a predictable way by using a central store and unidirectional data flow.
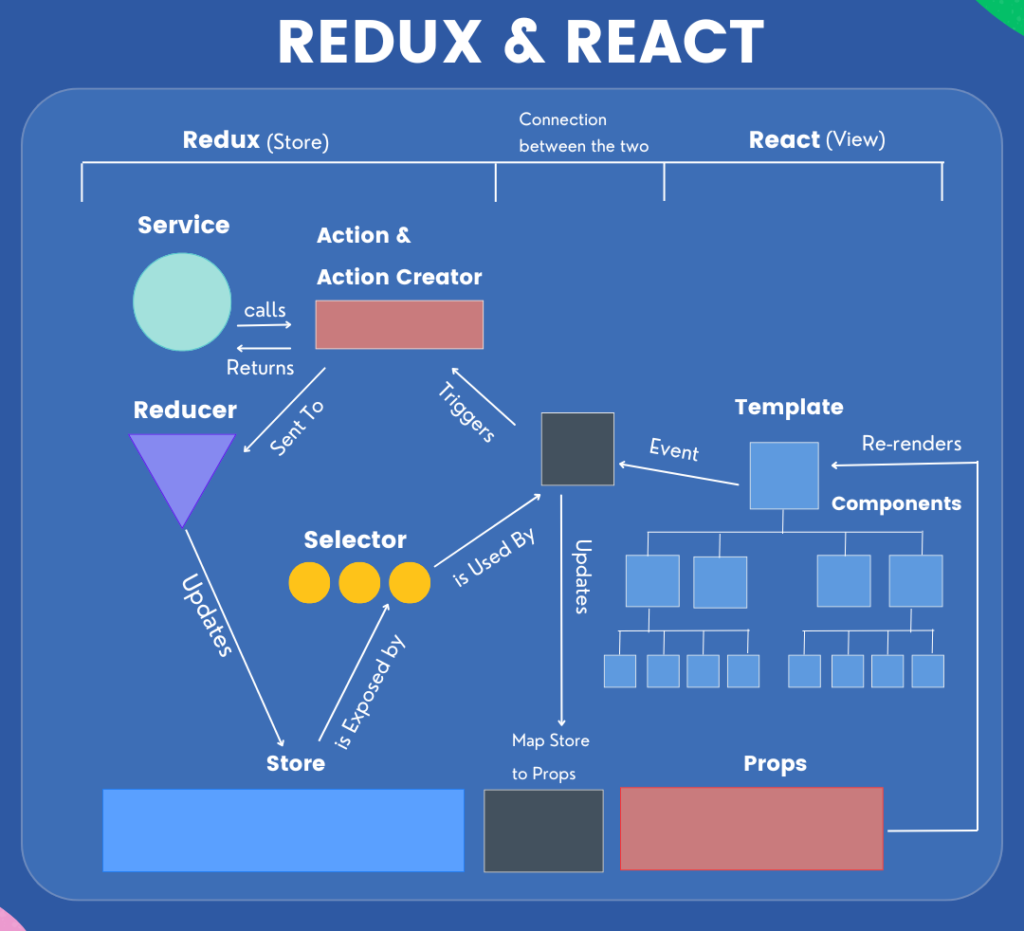
Introduction to Redux
Redux provides a centralized state container for managing application state. It follows three core principles:
- Single Source of Truth: The state of your whole application is stored in an object tree within a single store.
- State is Read-Only: The only way to change the state is to dispatch an action, an object describing what happened.
- Changes are Made with Pure Functions: To specify how the state tree is transformed by actions, you write pure reducers.
Setting up Redux in a React Project
To set up Redux in a React project, you need to install the required packages and configure the store.
Step 1: Install Redux and React-Redux
npm install redux react-redux
Step 2: Configure the Store
Create a Redux store and provide it to your React application.
Example:
// store.js
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
export default store;
Step 3: Provide the Store
Wrap your application with the Provider
component from react-redux
to make the store available to all components.
Example:
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Understanding Actions, Reducers, and Store
Actions
Actions are payloads of information that send data from your application to the Redux store. They are the only source of information for the store. Actions are plain JavaScript objects with a type
property that indicates the type of action being performed.
Example:
// actions.js
export const INCREMENT = 'INCREMENT';
export const DECREMENT = 'DECREMENT';
export const increment = () => ({
type: INCREMENT,
});
export const decrement = () => ({
type: DECREMENT,
});
Reducers
Reducers are functions that specify how the application’s state changes in response to actions. They are pure functions that take the current state and an action as arguments and return a new state.
Example:
// reducers.js
import { INCREMENT, DECREMENT } from './actions';
const initialState = {
count: 0,
};
function counterReducer(state = initialState, action) {
switch (action.type) {
case INCREMENT:
return {
...state,
count: state.count + 1,
};
case DECREMENT:
return {
...state,
count: state.count - 1,
};
default:
return state;
}
}
export default counterReducer;
Store
The store holds the entire state tree of your application. The only way to change the state inside the store is to dispatch an action to it. The store is created using the createStore
function from Redux.
Example:
// store.js
import { createStore } from 'redux';
import counterReducer from './reducers';
const store = createStore(counterReducer);
export default store;
Connecting React Components to Redux Store
To connect React components to the Redux store, you use the connect
function from react-redux
and the useSelector
and useDispatch
hooks.
Using connect
:
Example:
// Counter.js
import React from 'react';
import { connect } from 'react-redux';
import { increment, decrement } from './actions';
function Counter({ count, increment, decrement }) {
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
const mapStateToProps = (state) => ({
count: state.count,
});
const mapDispatchToProps = {
increment,
decrement,
};
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
Using useSelector
and useDispatch
Hooks:
Example:
// Counter.js
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement } from './actions';
function Counter() {
const count = useSelector((state) => state.count);
const dispatch = useDispatch();
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
}
export default Counter;
In this example, useSelector
is used to access the state from the Redux store, and useDispatch
is used to dispatch actions.
Conclusion
Redux provides a powerful way to manage the state of your React application. By setting up Redux, understanding actions, reducers, and the store, and connecting React components to the Redux store, you can build applications with predictable state management and a clear data flow. These principles help you maintain and scale your application effectively.