Contents
Control Structures
Decision Making in C (if , if..else, Nested if, if-else-if )
Conditional statements (also known as decision control structures) in C, such as if
, if-else
, and switch
, allow decision-making in programs by evaluating one or more conditions and directing the flow of program execution accordingly. They play a crucial role in determining which part of the code gets executed based on the evaluation of conditions.
Why Do We Need Conditional Statements?
In real-life scenarios, we often face situations where decisions need to be made based on certain conditions. Similarly, in programming, we use conditional statements to decide which block of code should be executed based on the evaluation of conditions. For example, in C, if condition x
is true, execute block A
; otherwise, execute block B
. In cases where multiple conditions exist, we use structures like if-else-if
or switch
to handle them efficiently.
Types of Conditional Statements in C
1. if Statement
2. if-else Statement
3. Nested if Statement
4. if-else-if Ladder
5. switch Statement
6. Conditional (Ternary) Operator
7. Jump Statements (break, continue, goto, return)
1. if
Statement in C : The if
statement is the simplest form of decision-making. It executes a block of code only when a specified condition is true.
Syntax:

Syntax:
if (condition) {
// Statements to execute if the condition is true
}
Example:
#include
int main() {
int x = 10;
if (x > 5) {
printf("x is greater than 5\n");
}
printf("Program continues...\n");
return 0;
}
Output:
x is greater than 5
Program continues...
2. if-else
Statement in C : The if-else
statement provides a choice between two blocks of code: one that is executed if the condition is true and another that is executed if the condition is false.

Syntax:
if (condition) {
// Executes if condition is true
} else {
// Executes if condition is false
}
Example:
#include
int main() {
int x = 8;
if (x > 10) {
printf("x is greater than 10\n");
} else {
printf("x is less than or equal to 10\n");
}
return 0;
}
Output:
x is less than or equal to 10
3. Nested if-else
Statement in C : A nested if-else
statement is an if
statement inside another if
statement. This allows for more complex decision-making.

Syntax:
if (condition1) {
if (condition2) {
// Executes if both condition1 and condition2 are true
} else {
// Executes if condition1 is true and condition2 is false
}
} else {
// Executes if condition1 is false
}
Example:
#include
int main() {
int x = 15;
if (x > 10) {
if (x < 20) {
printf("x is between 10 and 20\n");
} else {
printf("x is 20 or more\n");
}
} else {
printf("x is 10 or less\n");
}
return 0;
}
4. if-else-if
Ladder in C : The if-else-if
ladder is used to handle multiple conditions. It evaluates conditions from top to bottom, and the first condition that evaluates to true will have its associated block executed.

Syntax:
if (condition1) {
if (condition2) {
// Executes if both condition1 and condition2 are true
} else {
// Executes if condition1 is true and condition2 is false
}
} else {
// Executes if condition1 is false
}
Example:
#include
int main() {
int x = 15;
if (x > 10) {
if (x < 20) {
printf("x is between 10 and 20\n");
} else {
printf("x is 20 or more\n");
}
} else {
printf("x is 10 or less\n");
}
return 0;
}
5. switch
Statement in C : The switch
statement is an alternative to using multiple if-else
conditions. It allows for a variable to be compared with different values, and the corresponding block of code is executed.
Syntax:
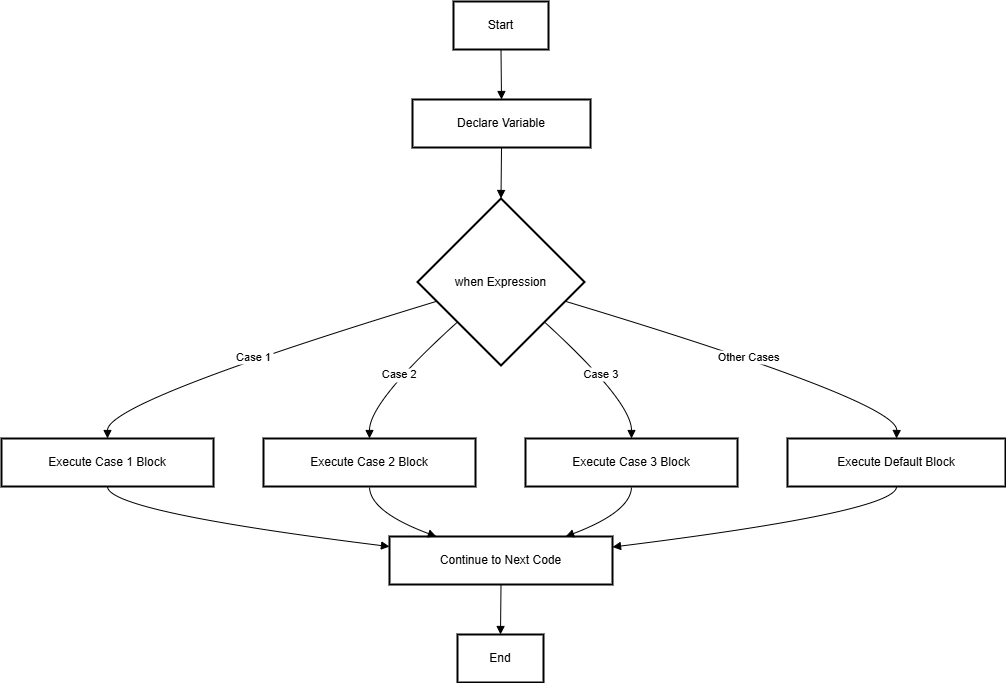
Syntax:
switch (expression) {
case value1:
// Statements for value1
break;
case value2:
// Statements for value2
break;
default:
// Default statements
}
Example:
Choice is 2
6. Conditional (Ternary) Operator in C : The conditional operator is a shorthand for the if-else
statement. It uses three operands to evaluate a condition and return one of two values based on whether the condition is true or false.
Syntax:
condition ? expression_if_true : expression_if_false;
Example:
#include
int main() {
int x = 5;
int result = (x > 0) ? 1 : 0;
printf("Result: %d\n", result);
return 0;
}
Output:
Result: 1
7. Jump Statements in C : Jump statements are used for unconditional flow of control. They include:
break
: Terminates a loop or switch statement.continue
: Skips the remaining code in the current iteration of a loop and starts the next iteration.goto
: Jumps to a labeled statement.return
: Exits the function and returns control to the caller.

Example of break
:
#include
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 6) {
break; // Terminates the loop when i is 6
}
printf("%d ", i);
}
return 0;
}
Output:
1 2 3 4 5
if Statement
The if
statement in C is a decision-making construct that allows you to execute a block of code based on a specified condition. It is one of the foundational concepts in C programming, enabling the inclusion of conditional logic to control the flow of the program.
Syntax:
if (condition) {
// Block of code to execute if the condition is true
}
Example:
Here is an example demonstrating the usage of the if
statement:
// C Program to demonstrate the usage of if statement
#include
int main() {
int num = 9;
// if statement with a true condition
if (num < 10) {
printf("%d is less than 10\n", num);
}
// if statement with a false condition
if (num > 20) {
printf("%d is greater than 20\n", num);
}
return 0;
}
Output:
9 is less than 10
Example:
In this example, we check whether a number is even or odd by using the modulus operator.
// C Program to check if a number is even or odd
#include
int main() {
int num = 4956;
// Check if the number is even
if (num % 2 == 0) {
printf("%d is Even\n", num);
}
// Else, the number is odd
else {
printf("%d is Odd\n", num);
}
return 0;
}
Output:
4956 is Even
if…else Statement
The if-else
statement in C is a control flow mechanism used to make decisions in a program. It extends the basic if
statement by adding an else
block, allowing for the execution of an alternative set of statements when the if
condition evaluates to false.
if (condition) {
// code executed when the condition is true
}
Example:
The following program demonstrates how the if-else
statement works in C:
// C Program to demonstrate the use of if-else statement
#include
int main() {
// if block with condition
if (5 < 10) {
// will be executed if the condition is true
printf("5 is less than 10.");
}
// else block
else {
// will be executed if the condition is false
printf("5 is greater than 10.");
}
return 0;
}
Output:
5 is less than 10.
if-else-if Statement
The if-else-if
ladder in C is a sequential structure used to test multiple conditions one after the other. If any condition evaluates to true
, the corresponding block of code will execute, and the ladder will terminate. The conditions are tested only if all the preceding if
or else-if
conditions are false
.
Syntax:
if (condition) {
// code to execute if condition is true
}
else if (condition) {
// executed if the first condition is false
// and this condition is true
}
... // multiple else-if blocks can follow
else {
// executed if all above conditions are false
}
Output:
Using Prefix Increment on x = 8
Using Postfix Increment on y = 7
Working Flow of the if-else-if Ladder:
1. The program first enters the if block.
2. The first condition is evaluated:
- If the condition is true, the corresponding code block is executed, and the ladder terminates.
- If the condition is false, the program moves to the next else-if block.
3. The next else-if condition is evaluated:
- If the condition is true, its associated block is executed, and the ladder terminates.
- If the condition is false, the process continues with subsequent else-if blocks.
4. If none of the if or else-if conditions are true, the final else block is executed.
5. The program exits the if-else-if ladder after execution of the appropriate block.
Example: Check Whether a Number is Positive, Negative, or Zero
In the following example, we check if a number is positive, negative, or zero using the if-else-if ladder:
// C Program to check whether a number is positive, negative, or zero
#include
int main() {
int number = 0;
// Condition for positive numbers
if (number > 0) {
printf("The number is Positive\n");
}
// Condition for negative numbers
else if (number < 0) {
printf("The number is Negative\n");
}
// Condition for zero
else {
printf("The number is Zero\n");
}
return 0;
}
Output:
The number is Zero
Example of Decrement Operator:
#include
int main() {
int a = 9;
int b = 9;
printf("Using Prefix Decrement on a = %d\n", --a);
printf("Using Postfix Decrement on b = %d", b--);
return 0;
}
Output:
Using Prefix Decrement on a = 8
Using Postfix Decrement on b = 9
Switch Statement
The switch case statement in C is used to evaluate an expression and execute different blocks of code based on the matched case. It allows control to be transferred to various parts of a program depending on the evaluated value, making it an effective alternative to multiple if
statements.
How Switch Case Works
The switch case statement follows a selection-control mechanism, where the value of a given expression determines which case block should execute. This can be particularly useful for simplifying long sequences of if-else
statements that compare a variable to multiple possible values.
Syntax of Switch Statement in C
switch (expression) {
case value1:
// code to execute when expression equals value1
break;
case value2:
// code to execute when expression equals value2
break;
...
case value_n:
// code to execute when expression equals value_n
break;
default:
// code to execute if none of the cases match
}
How to Use Switch Case in C
Before using switch case, it’s important to understand the following rules:
- The “case value” must be of
char
orint
type. - You can have one or more cases.
- Each case must have a unique value.
- A
break
statement is optional but commonly used to prevent fall-through. - The
default
case is also optional but is used to handle values that don’t match any case.
Example:
// C program to demonstrate the use of switch case statement
#include
int main() {
int day = 3;
switch (day) {
case 1:
printf("Sunday");
break;
case 2:
printf("Monday");
break;
case 3:
printf("Tuesday");
break;
default:
printf("Invalid day");
}
return 0;
}
How the Switch Statement Works
Step 1: The expression in the switch statement is evaluated.
Step 2: The value of the expression is compared with each case.
Step 3A: If a matching case is found, the associated block is executed.
Step 3B: If no matching case is found, the default case is executed (if present).
Step 4A: If the break keyword is present, the program exits the switch block.
Step 4B: Without break, execution falls through to the next case.
Step 5: The program continues executing the code after the switch statement.
Example: Switch Case Without Break
// C program to demonstrate behavior without break
#include
int main() {
int var = 2;
switch (var) {
case 1:
printf("Case 1 executed\n");
case 2:
printf("Case 2 executed\n");
case 3:
printf("Case 3 executed\n");
default:
printf("Default case executed\n");
}
return 0;
}
Output:
Case 2 executed
Case 3 executed
Default case executed
Relational Operators in C
In C programming, loops are essential for performing repetitive tasks efficiently. The for
loop allows a block of code to be executed repeatedly based on a given condition. It is a type of entry-controlled loop that is widely used for iterating through arrays, strings, and other data structures.
What is the for
Loop in C?
The for
loop in C is a control structure that repeats a block of statements a specified number of times. It is different from other loops like while
and do-while
because it contains initialization, condition checking, and updating statements all in one line.
Syntax of for
Loop
for (initialization; condition; update) {
// code to be executed repeatedly
}
Structure of for
Loop:
1. Initialization: Initializes the loop control variable. This step is executed only once, at the beginning of the loop.
2. Condition: This is the condition that is checked before each iteration of the loop. If the condition is true, the loop continues; otherwise, it terminates.
3. Body: The block of statements that is executed repeatedly as long as the condition is true.
4. Update: This is where the loop control variable is updated, usually by incrementing or decrementing, after every iteration.
How the for
Loop Works
Step 1: Initialization is done at the start of the loop.
Step 2: The condition is evaluated. If it is true, the loop body is executed.
Step 3: After the body is executed, the loop control variable is updated.
Step 4: The loop repeats from Step 2, continuing until the condition becomes false.
Example of for
Loop
// C program to demonstrate for loop
#include
int main() {
int gfg;
// for loop with initialization, condition, and update
for (gfg = 1; gfg <= 5; gfg++) {
printf("HelloWorld\n");
}
return 0;
}
Output:
HelloWorld
HelloWorld
HelloWorld
HelloWorld
HelloWorld
while Loop
The while
loop in C is an entry-controlled loop that executes a block of code repeatedly as long as the given condition remains true. It is one of the most common loops used in C programming.

Syntax of while
Loop
while (test expression) {
// code block consisting of multiple statements
}
Example of while
Loop
The following example demonstrates how to use a while
loop in C:
// C program to demonstrate while loop
#include
int main() {
// Initialization of loop variable
int i = 0;
// The loop will run while i is less than 5
while (i < 5) {
// Loop body
printf("Hello, World\n");
// Incrementing the loop variable
i++;
}
return 0;
}
Output:
Hello, World
Hello, World
Hello, World
Hello, World
Hello, World
Structure of while
Loop
The while
loop in C follows a structured approach, consisting of the following parts:
1. Initialization: Before the loop begins, a loop control variable is initialized. This step is essential for controlling the loop but is not part of the loop’s syntax.
2. Conditional Statement: This step checks whether the loop should continue. The loop body executes only if the condition is true. Once the condition becomes false, the loop terminates.
3. Body: The actual block of code that is executed as long as the condition is true. It is typically enclosed within curly braces {}.
4. Updation: After each iteration, the loop variable is updated (usually incremented or decremented).
How the while Loop Works
Step 1: The loop starts by evaluating the test condition.
Step 2A: If the condition is false, the loop terminates and control passes to the next part of the program.
Step 2B: If the condition is true, the loop body executes.
Step 3: After executing the loop body, control returns to Step 1 for the next iteration. This process continues until the condition becomes false.
do while Loop
In C programming, loops are control flow statements that allow a section of code to be executed repeatedly as long as a specified condition is true. Among the three types of loops in C—the do-while
loop, while
loop, and for
loop—the do-while
loop is particularly useful for iterating through arrays, vectors, and other data structures.

Syntax of do...while
Loop in C
do {
// loop body (statements to execute)
} while (condition);
Example:
// C Program to demonstrate do...while loop behavior
// when the condition is false from the start
#include
#include
int main() {
// Declaring a false condition
bool condition = false;
do {
printf("This is the loop body.\n");
} while (condition); // false condition
return 0;
}
Output:
This is the loop body.
Structure of while
Loop
The do-while loop in C has a structured format with the following components:
1. Initialization: A loop control variable is initialized before the loop begins. This step is crucial for controlling the loop but is not part of the loop’s syntax.
2. Body: The main block of code to be executed is placed here. Unlike other loops, the body of the do-while loop runs at least once before the condition is checked. The code block is typically enclosed within curly braces {}.
3. Conditional Statement: After executing the loop body, the loop checks a condition. If the condition is true, the loop repeats from the start of the body. When the condition becomes false, the loop terminates.
4. Updation: Within the loop body, the control variable is usually updated (e.g., incremented or decremented) to progress toward the condition becoming false.
How the do while Loop Works
Step 1: The loop begins by executing the body of the loop at least once, regardless of the condition.
Step 2: After the loop body executes, the test condition is evaluated.
Step 2A: If the condition is false, the loop terminates, and control moves to the next part of the program.
Step 2B: If the condition is true, control returns to Step 1 to repeat the loop body.
Step 3: This process continues until the condition evaluates to false.