Contents
PHP Loops
PHP Loops
Similar to other programming languages, loops in PHP are used to repeatedly execute a block of code multiple times based on a condition. PHP offers several types of loops to handle different situations, including for
, while
, do...while
, and foreach
loops. Let’s explore each of these loops, their syntax, and examples.
Why Use Loops?
Loops are useful for executing code repeatedly, which helps in:
- Iterating through arrays or other data structures.
- Performing actions multiple times.
- Pausing execution until a condition is satisfied.
1. PHP for
Loop: The for
loop is used when you know in advance how many times you want to execute a block of code. It consists of three parts:
- Initialization: Sets the initial value of the loop variable.
- Condition: Evaluates whether the loop should continue.
- Increment/Decrement: Updates the loop variable after each iteration.
Syntax:
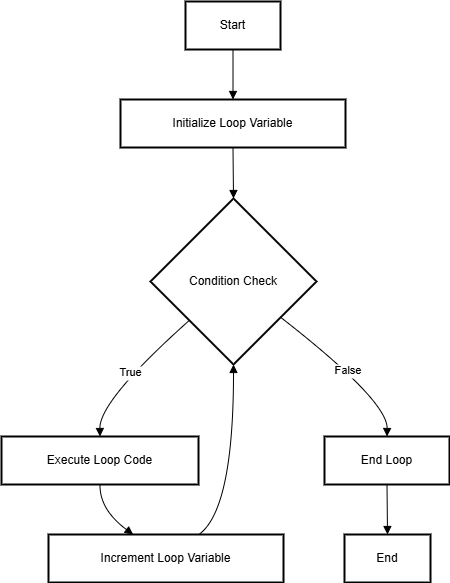
for (Initialization; Condition; Increment/Decrement) {
// Code to execute
}
Example:
Output:
10 11 12 13 14 15
2. PHP while
Loop: The while
loop is an entry-controlled loop, meaning it first checks the condition before entering the loop. It continues running as long as the condition remains true.
Syntax:
while (condition) {
// Code to execute
}
Example: Printing numbers from 5 to 9.
Output:
5 6 7 8 9
3. PHP do...while
Loop: The do...while
loop is an exit-controlled loop. It executes the code block first and then checks the condition, which means the code will run at least once, regardless of the condition.
Syntax:
do {
// Code to execute
} while (condition);
Example:
Output:
20 21 22 23 24 25
4. PHP foreach
Loop: The foreach
loop is used to iterate over arrays. For each iteration, it assigns the current array element to a variable and moves on to the next one.
Syntax:
foreach ($array as $value) {
// Code to execute
}
// or
foreach ($array as $key => $value) {
// Code to execute
}
Example:
50,
"Banana" => 30,
"Orange" => 20
);
foreach ($fruits as $fruit => $price) {
echo $fruit . " => " . $price . "\n";
}
?>
Output:
100 200 300 400
Apple => 50
Banana => 30
Orange => 20
PHP while Loop
The while
loop is one of the simplest looping structures in PHP, used to repeatedly execute a block of code as long as the condition evaluates to true. The condition is evaluated at the start of each iteration, and if it’s true, the code block is executed. If the condition becomes false, the loop terminates.
Syntax:
while (condition) {
// Code to be executed
}
Example 1: Using a while
loop to display even numbers from 2 to 8.
Output:
2
4
6
8
while-endwhile
Loop
In PHP, there’s an alternate syntax for the while
loop known as the while-endwhile
structure. This style is often used in PHP templating to improve readability.
Syntax:
while (condition):
// Code to execute
endwhile;
Example 2: Using the while-endwhile
loop to display odd numbers from 3 to 9.
Output:
3
5
7
9
PHP do-while Loop
The do-while
loop is quite similar to the while
loop, with the key difference being that the do-while
loop checks the condition at the end of each iteration. This means that the block of code inside the do-while
loop is executed at least once, even if the condition is initially false.
do {
// Code to execute
} while (condition);
Example 1: Displaying numbers starting from 15, incrementing by 3 until the number is less than 25.
Output:
15
18
21
24
Example 2: Adding 7 to a number in each iteration until the number exceeds 30.
Output:
8
15
22
29
36
PHP for Loop
The for
loop in PHP is a powerful and flexible control structure, typically used when the number of iterations is known in advance. The loop involves three main expressions: initialization, condition testing, and updating the loop variable (increment or decrement).
for (initialization expression; test condition; update expression) {
// Code to be executed
}
Parameters of the for
Loop:
1. Initialization Expression: This sets the starting value of the loop control variable. For example: $num = 0;.
2. Test Condition: This condition is evaluated at the start of each loop iteration. If it returns true, the loop body is executed; if it returns false, the loop terminates. For example: $num < 10;.
3. Update Expression: After each loop iteration, the control variable is updated (usually incremented or decremented). For example: $num += 2;.
Example:
Output:
2
6
10
14
18
Example 2: Printing numbers divisible by 6 between 1 and 36.
Output:
6
12
18
24
30
36
PHP foreach Loop
The foreach
loop in PHP is designed specifically for working with arrays and objects. Unlike other loops, such as for
or while
, it automatically iterates over each element of the array or object without the need for manually managing indices or counters, making the code more readable and less error-prone.
Key Benefits of foreach
Loop:
- Simple and clean syntax.
- Automatically handles both keys and values in associative arrays.
- Reduces the need for manual index management.
Syntax of foreach
Loop:
foreach( $array as $element ) {
// Code to be executed
}
Or, to access both keys and values:
foreach( $array as $key => $element ) {
// Code to be executed
}
Examples of foreach
Loop:
1. Iterating through Indexed Arrays: Indexed arrays contain elements that are stored with numeric keys. The foreach
loop can iterate over them easily.
Output:
5
10
15
20
25
2. Iterating through Associative Arrays: Associative arrays store elements in key-value pairs. The foreach
loop can handle both the key and the value, making it ideal for these types of arrays.
"john_doe",
"age" => 28,
"email" => "john.doe@example.com"
];
foreach ($user as $attribute => $detail) {
echo "$attribute: $detail" . PHP_EOL;
}
?>
Output:
username: john_doe
age: 28
email: john.doe@example.com
3. Iterating through Multidimensional Arrays: A multidimensional array contains other arrays as its elements. The foreach
loop can be nested to handle multidimensional arrays.
"Laptop", "price" => 1200],
["name" => "Smartphone", "price" => 800],
["name" => "Tablet", "price" => 400]
];
foreach ($products as $product) {
foreach ($product as $key => $value) {
echo "$key: $value" . PHP_EOL;
}
echo "-----" . PHP_EOL;
}
?>
Output:
name: Laptop
price: 1200
-----
name: Smartphone
price: 800
-----
name: Tablet
price: 400
-----
4. Iterating through Object Properties: The foreach
loop can also be used to iterate through the properties of an object. This is useful when dealing with data stored in objects.
title = $title;
$this->author = $author;
$this->year = $year;
}
}
$book = new Book("1984", "George Orwell", 1949);
foreach ($book as $property => $value) {
echo "$property: $value" . PHP_EOL;
}
?>
Output:
title: 1984
author: George Orwell
year: 1949
5. Using break
and continue
in foreach
Loop: The break
statement exits the loop, while continue
skips the current iteration and proceeds to the next one.
Output:
2
4
6