Contents
Introduction to JavaScript
JavaScript is a versatile, high-level programming language that is an essential part of web development. It allows developers to create dynamic and interactive web content, making websites more engaging and functional. This introduction will cover what JavaScript is, its importance in web development, basic syntax, and how to add JavaScript to HTML.
What is JavaScript?
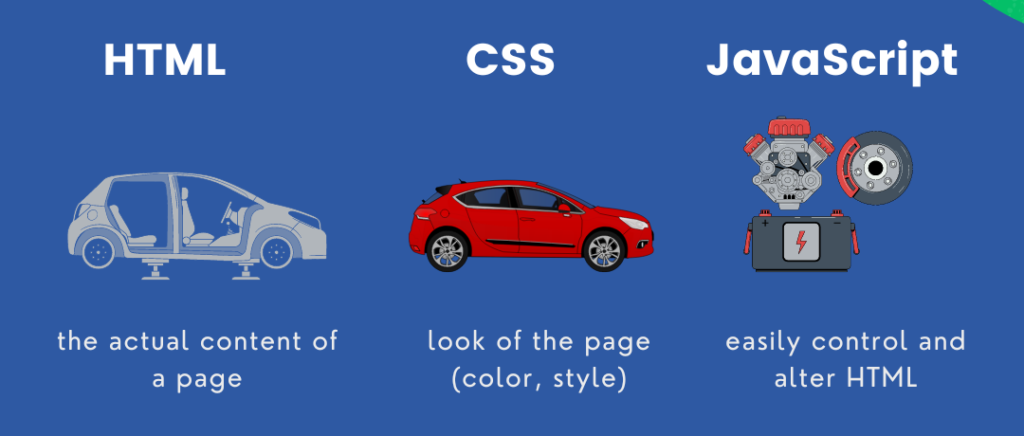
JavaScript is a client-side scripting language primarily used to enhance the interactivity of websites. It allows developers to implement complex features such as interactive forms, animations, and dynamic content updates without needing to reload the web page.
Key Features of JavaScript:
- Dynamic Content: JavaScript can change and update both HTML and CSS to create dynamic content, such as displaying live weather updates or interactive image sliders.
- Interactivity: It allows you to respond to user events like clicks, form submissions, and keyboard input.
- Cross-Platform: Works in all major web browsers, making it widely accessible.
- Versatile: Can be used for both front-end (in the browser) and back-end (e.g., using Node.js) development.
Importance of JavaScript in Web Development
JavaScript is one of the core technologies of the web, alongside HTML and CSS. Here’s why it’s crucial for modern web development:
2.1 Enhances User Experience
JavaScript enables the creation of interactive elements, such as:
- Forms: Validate form inputs in real-time, enhancing usability.
- Animations: Create animated elements like carousels and modals.
- Dynamic Content: Load content dynamically without refreshing the page using AJAX or Fetch API.
2.2 Enables Rich User Interfaces
Frameworks and libraries like React, Vue, and Angular are built on JavaScript, helping developers build complex user interfaces efficiently.
2.3 Full-Stack Development
JavaScript can be used on both the client side (browser) and the server side (Node.js), enabling full-stack development with a single language.
Basic JavaScript Syntax
JavaScript syntax consists of various elements like variables, data types, operators, functions, and control structures. Here’s an overview of the basics.
3.1 Variables
Variables are used to store data values. In modern JavaScript, you can declare variables using let
, const
, or var
.
let
: Declares a block-scoped variable.
let name = "John";
let age = 25;
const
: Declares a constant, which means its value cannot be changed.
const pi = 3.14;
var
: An older way to declare variables, with function scope.
var city = "New York";
3.2 Data Types
JavaScript has several data types:
- String: Text enclosed in quotes.
let greeting = "Hello, World!";
- Number: Numeric values.
let count = 10;
- Boolean: Represents logical values (
true
orfalse
).
let isStudent = true;
- Array: A collection of values.
let colors = ["red", "green", "blue"];
- Object: A collection of key-value pairs.
let person = {
name: "Alice",
age: 30
};
3.3 Operators
JavaScript supports arithmetic, comparison, and logical operators.
- Arithmetic Operators:
+
,-
,*
,/
,%
let sum = 10 + 5; // 15
- Comparison Operators:
==
,===
,!=
,!==
,<
,>
,<=
,>=
let isEqual = (5 === 5); // true
- Logical Operators:
&&
(AND),||
(OR),!
(NOT)
let isAdult = (age >= 18 && age <= 65);
3.4 Functions
Functions are blocks of reusable code that perform a specific task.
function greet(name) {
return "Hello, " + name + "!";
}
console.log(greet("Alice")); // Output: Hello, Alice!
Adding JavaScript to HTML
JavaScript can be added to an HTML document in three main ways: inline, internal, and external.
4.1 Inline JavaScript
You can include JavaScript directly within an HTML element using the onclick
attribute (or other event attributes).
4.2 Internal JavaScript
Place JavaScript code within the <script>
tag inside the HTML document. This is useful for small scripts or testing purposes.
Internal JavaScript
Welcome to JavaScript
4.3 External JavaScript
The best practice is to write JavaScript in a separate file and link it to your HTML document. This keeps your code organized and reusable.
Step 1: Create an external JavaScript file, e.g.,
script.js
.
// script.js
console.log("External JavaScript file linked!");
- Step 2: Link the JavaScript file to your HTML document using the
<script>
tag with thesrc
attribute.
External JavaScript
External JavaScript Example
- Placement: It’s generally recommended to place the
<script>
tag before the closing</body>
tag to ensure the HTML content is fully loaded before the JavaScript executes.
Summary
JavaScript is a versatile, essential programming language for web development. It enables you to create dynamic and interactive user experiences by manipulating HTML and CSS on the fly. JavaScript’s syntax includes variables, data types, operators, and functions, making it a powerful tool for building complex web applications. You can integrate JavaScript into your HTML using inline, internal, or external scripts, with external scripts being the best practice for maintainability and reusability. By mastering JavaScript, you unlock the ability to create engaging, user-friendly websites.