Contents
Objects and Arrays
Objects and arrays are foundational data structures in JavaScript. Objects allow you to store related data and functions, while arrays let you hold an ordered list of values. JavaScript provides a robust set of methods for manipulating both, making them versatile tools in programming.
Objects
Objects in JavaScript are collections of key-value pairs, where keys (also called properties) are strings (or symbols) and values can be any data type, including other objects, arrays, or functions.
1.1 Creating Objects
You can create objects using the object literal syntax or the new Object()
syntax.
Object Literal:
const person = {
name: "Alice",
age: 30,
isStudent: false,
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
- Using
new Object()
:
const person = new Object();
person.name = "Alice";
person.age = 30;
1.2 Accessing and Modifying Object Properties
Dot Notation: Access properties using the dot
.
operator.
console.log(person.name); // Output: "Alice"
person.age = 31;
- Bracket Notation: Use brackets
[]
to access properties, useful for keys with special characters or when using variables.
console.log(person["age"]); // Output: 31
const key = "name";
console.log(person[key]); // Output: "Alice"
1.3 Adding and Deleting Properties
Adding Properties:
person.email = "alice@example.com";
- delete person.age;
delete person.age;
1.4 Nested Objects
Objects can contain other objects, enabling the representation of complex data.
const user = {
name: "John",
contact: {
email: "john@example.com",
phone: "123-456-7890"
}
};
console.log(user.contact.email); // Output: "john@example.com"
Arrays
Arrays are ordered collections of values (elements) and can contain any data type, including numbers, strings, objects, and even other arrays.
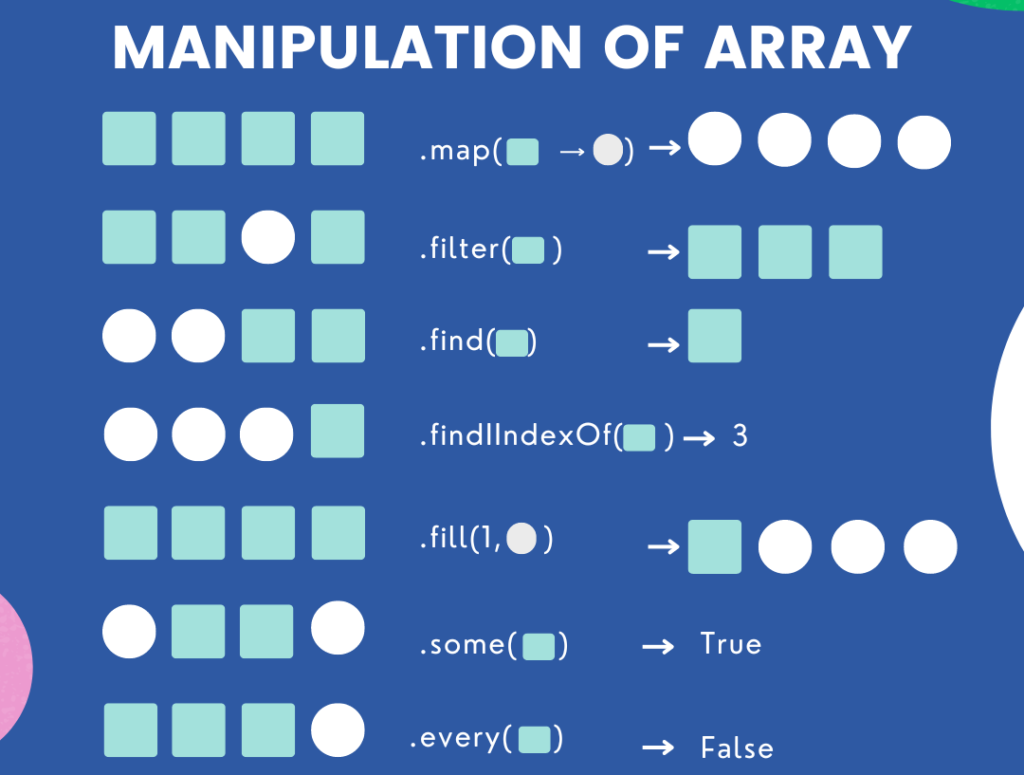
2.1 Creating Arrays
Array Literal: The most common way to create an array.
const colors = ["red", "green", "blue"];
- Using
new Array()
:
const numbers = new Array(1, 2, 3, 4, 5);
2.2 Accessing and Modifying Array Elements
Accessing Elements: Use the index (starting at 0) to access elements.
console.log(colors[0]); // Output: "red"
- Modifying Elements:
colors[1] = "yellow";
console.log(colors); // Output: ["red", "yellow", "blue"]
2.3 Common Array Properties
length
: Returns the number of elements in the array.
console.log(colors.length); // Output: 3
Array Methods
JavaScript provides numerous built-in methods to manipulate arrays, making it easier to handle data. Here are some commonly used array methods:
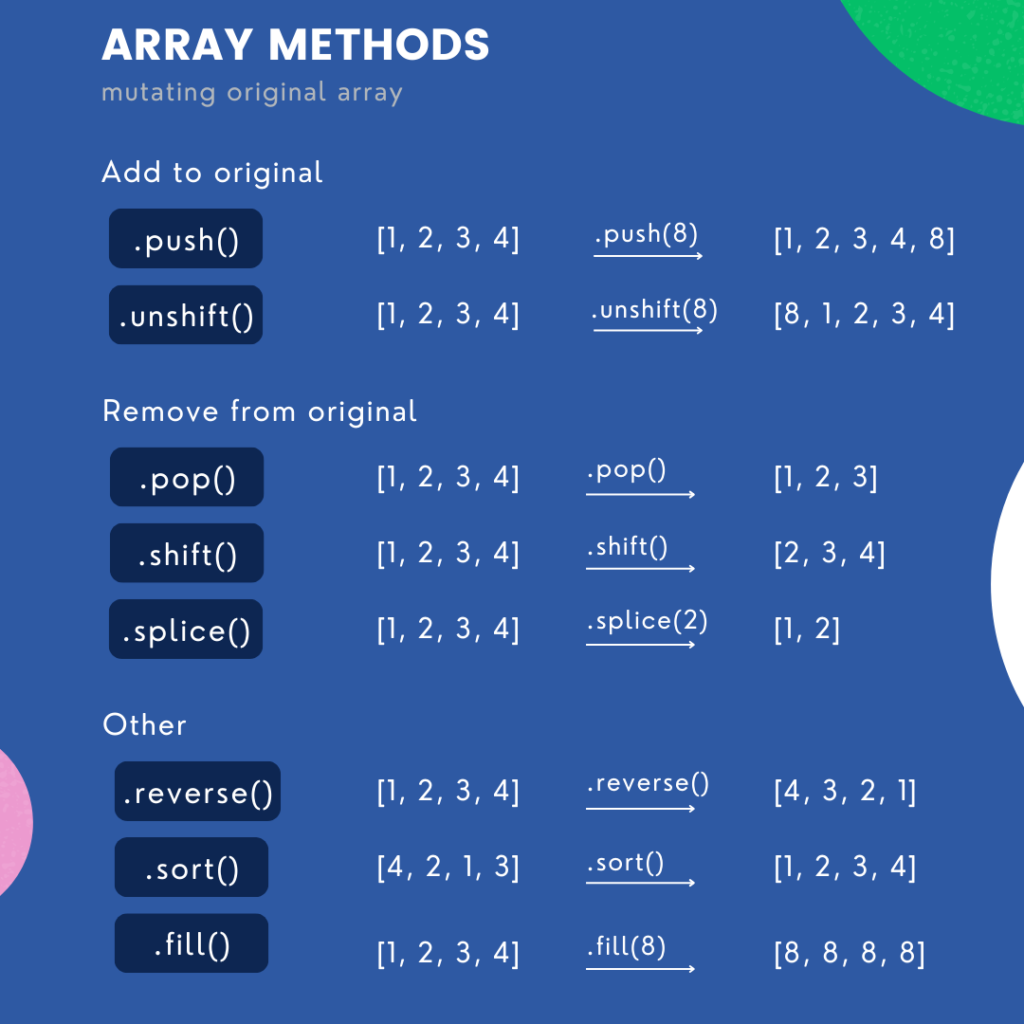
3.1 Adding and Removing Elements
push()
: Adds one or more elements to the end of an array.
colors.push("purple");
console.log(colors); // Output: ["red", "yellow", "blue", "purple"]
pop()
: Removes the last element from an array and returns it.
const lastColor = colors.pop();
console.log(lastColor); // Output: "purple"
console.log(colors); // Output: ["red", "yellow", "blue"]
unshift()
: Adds one or more elements to the beginning of an array.
colors.unshift("orange");
console.log(colors); // Output: ["orange", "red", "yellow", "blue"]
shift()
: Removes the first element from an array and returns it.
const firstColor = colors.shift();
console.log(firstColor); // Output: "orange"
console.log(colors); // Output: ["red", "yellow", "blue"]
3.2 Manipulating Arrays
splice()
: Adds or removes elements from an array.
colors.splice(1, 0, "green"); // Inserts "green" at index 1
console.log(colors); // Output: ["red", "green", "yellow", "blue"]
slice()
: Returns a shallow copy of a portion of an array into a new array.
const newColors = colors.slice(1, 3);
console.log(newColors); // Output: ["green", "yellow"]
3.3 Iterating Over Arrays
forEach()
: Executes a provided function once for each array element.
colors.forEach((color) => {
console.log(color);
});
map()
: Creates a new array populated with the results of calling a provided function on every element in the array.
const upperColors = colors.map(color => color.toUpperCase());
console.log(upperColors); // Output: ["RED", "GREEN", "YELLOW", "BLUE"]
filter()
: Creates a new array with all elements that pass a test implemented by the provided function.
const longColors = colors.filter(color => color.length > 4);
console.log(longColors); // Output: ["yellow"]
reduce()
: Executes a reducer function on each element of the array, resulting in a single output value.
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // Output: 10
3.4 Searching and Sorting Arrays
find()
: Returns the value of the first element that satisfies the provided testing function.
const foundColor = colors.find(color => color === "green");
console.log(foundColor); // Output: "green"
includes()
: Determines whether an array contains a certain value.
const hasBlue = colors.includes("blue");
console.log(hasBlue); // Output: true
sort()
: Sorts the elements of an array in place and returns the sorted array.
colors.sort();
console.log(colors); // Output: ["blue", "green", "red", "yellow"]
reverse()
: Reverses the order of the elements in an array in place.
colors.reverse();
console.log(colors); // Output: ["yellow", "red", "green", "blue"]
Summary
Objects in JavaScript store data as key-value pairs, allowing you to model real-world entities with properties and behaviors. Arrays store ordered lists of values, making them ideal for managing collections of data. JavaScript provides a wide range of array methods (push()
, pop()
, map()
, filter()
, etc.) to manipulate and interact with arrays efficiently. Understanding how to use objects, arrays, and their associated methods is essential for handling complex data in JavaScript applications.