Handling Events
Handling events in React is straightforward and similar to handling events in plain JavaScript. However, there are some key differences and benefits that come with React’s event system.
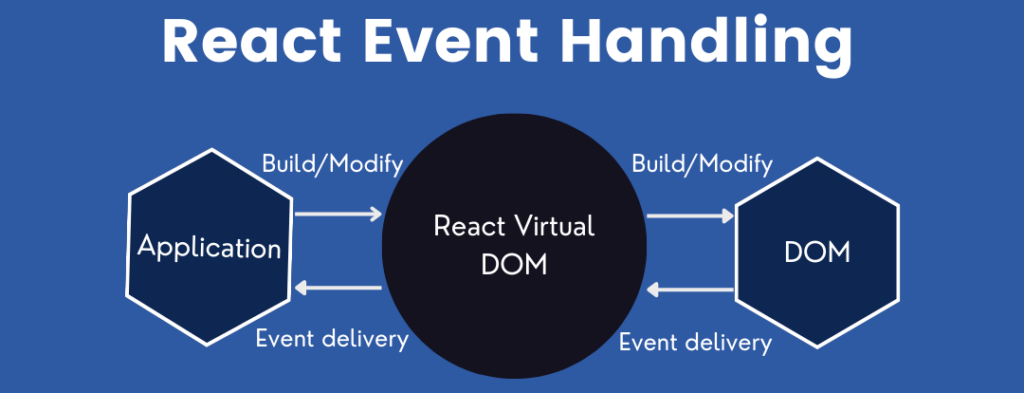
Adding Event Handlers in JSX
In React, you add event handlers directly in the JSX by using camelCase syntax for event names and passing a function as the event handler.
Example:
function ActionButton() {
function handleClick() {
alert('Button clicked!');
}
return <button onClick={handleClick}>Click Me</button>;
}
ReactDOM.render(<ActionButton />, document.getElementById('root'));
In this example, the handleClick
function is called when the button is clicked.
Synthetic Events in React
React normalizes events so that they have consistent properties across different browsers. This is achieved through a system called synthetic events. Synthetic events are wrappers around the native browser events and work identically across all browsers.
Example:
function MouseTracker() {
function handleMouseOver(event) {
console.log('Mouse over:', event.clientX, event.clientY);
}
return <div onMouseOver={handleMouseOver}>Hover over me!</div>;
}
ReactDOM.render(<MouseTracker />, document.getElementById('root'));
In this example, handleMouseOver
logs the mouse coordinates whenever the mouse is moved over the div.
Passing Arguments to Event Handlers
Sometimes, you need to pass additional arguments to your event handlers. You can achieve this by using an arrow function or the bind
method.
Using Arrow Functions:
Example:
function ActionButton() {
function handleClick(message) {
alert(message);
}
return (
<button onClick={() => handleClick('Button clicked!')}>
Click Me
</button>
);
}
ReactDOM.render(<ActionButton />, document.getElementById('root'));
In this example, an arrow function is used to call handleClick
with the message
argument.
Using bind
Method:
Example:
function ActionButton() {
function handleClick(message, event) {
alert(message);
console.log('Event:', event);
}
return (
<button onClick={handleClick.bind(this, 'Button clicked!')}>
Click Me
</button>
);
}
ReactDOM.render(<ActionButton />, document.getElementById('root'));
In this example, the bind
method is used to pass the message
argument to handleClick
. The event object is automatically passed as the second argument.
Conclusion
Handling events in React is a crucial aspect of creating interactive and dynamic applications. By understanding how to add event handlers in JSX, leverage synthetic events for cross-browser consistency, and pass arguments to event handlers, you can enhance the functionality of your React components. These skills form the foundation for building more complex user interactions in your React applications.
Next, we’ll explore how to handle forms in React, including controlled components, form submissions, and accessing DOM elements using refs.