Rendering Elements
Rendering elements is one of the core aspects of React, as it allows you to display dynamic content in your application. In this section, we’ll cover the basics of rendering a React component, updating rendered elements, and implementing conditional rendering.
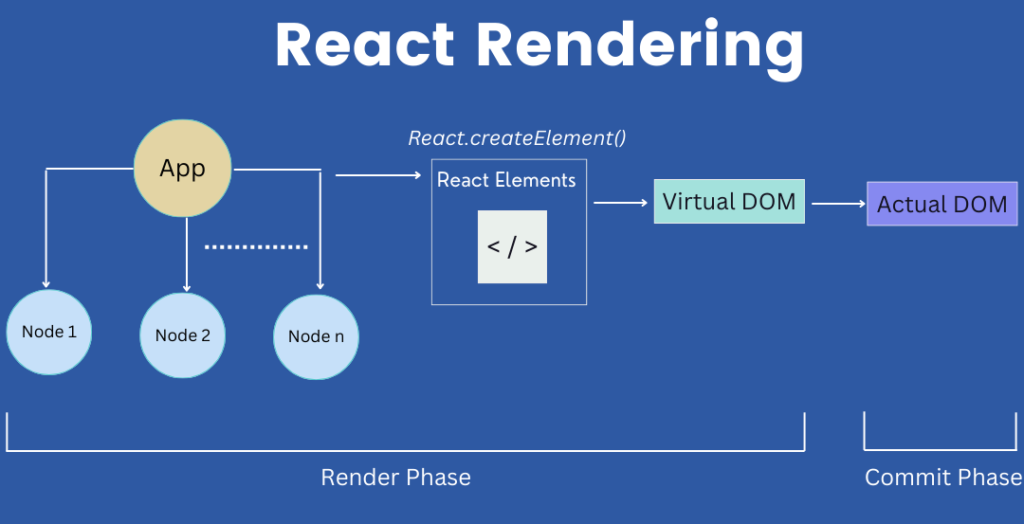
Rendering a React Component
To render a React component, you use the ReactDOM.render
method. This method takes two arguments: the component to render and the DOM element where the component should be mounted.
Here’s an example of rendering a simple Greeting
component:
Greeting Component:
// Greeting.js
import React from 'react';
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default Greeting;
Rendering the Component:
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import Greeting from './Greeting';
ReactDOM.render(<Greeting name="Niraj" />, document.getElementById('root'));
In this example, the Greeting
component is rendered into the DOM element with the ID root
.
Updating the Rendered Element
React efficiently updates the rendered elements using a virtual DOM. When the state or props of a component change, React updates only the parts of the DOM that have changed.
Here’s an example demonstrating how updating the state updates the rendered element:
Counter Component:
// Counter.js
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
Rendering the Component:
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import Counter from './Counter';
ReactDOM.render(<Counter />, document.getElementById('root'));
In this example, clicking the “Increment” button updates the count
state, and React re-renders the Counter
component with the new count value.
Conditional Rendering in React
Conditional rendering allows you to render different components or elements based on certain conditions. This can be achieved using JavaScript conditional operators within JSX.
Using if-else
for Conditional Rendering:
You can use JavaScript if-else
statements to conditionally render elements.
Example:
function UserGreeting(props) {
return <h1>Welcome back!</h1>;
}
function GuestGreeting(props) {
return <h1>Please sign up.</h1>;
}
function Greeting(props) {
const isLoggedIn = props.isLoggedIn;
if (isLoggedIn) {
return <UserGreeting />;
}
return <GuestGreeting />;
}
ReactDOM.render(<Greeting isLoggedIn={true} />, document.getElementById('root'));
Using Ternary Operator for Conditional Rendering:
The ternary operator is a more concise way to conditionally render elements.
Example:
function Greeting(props) {
const isLoggedIn = props.isLoggedIn;
return (
<div>
{isLoggedIn ? <h1>Welcome back!</h1> : <h1>Please sign up.</h1>}
</div>
);
}
ReactDOM.render(<Greeting isLoggedIn={false} />, document.getElementById('root'));
Using Logical &&
Operator for Conditional Rendering:
The logical &&
operator can be used to conditionally include an element.
Example:
function Mailbox(props) {
const unreadMessages = props.unreadMessages;
return (
<div>
<h1>Hello!</h1>
{unreadMessages.length > 0 && (
<h2>You have {unreadMessages.length} unread messages.</h2>
)}
</div>
);
}
const messages = ['React', 'Re: React', 'Re:Re: React'];
ReactDOM.render(
<Mailbox unreadMessages={messages} />,
document.getElementById('root')
);
In this example, the message count is displayed only if there are unread messages.
Conclusion
Rendering elements is a fundamental concept in React that enables you to create dynamic and interactive UIs. By understanding how to render components, update rendered elements, and implement conditional rendering, you can build more complex and responsive applications. React’s ability to efficiently update and render only the necessary parts of the DOM makes it a powerful tool for building modern web applications. Next, we’ll delve into more advanced concepts such as handling events and forms in React.