Contents
Understanding JSX
JSX is a syntax extension for JavaScript that combines the expressiveness of JavaScript with the familiarity of HTML. It is a core concept in React and makes it easier to create and visualize the structure of your user interfaces.
What is JSX?
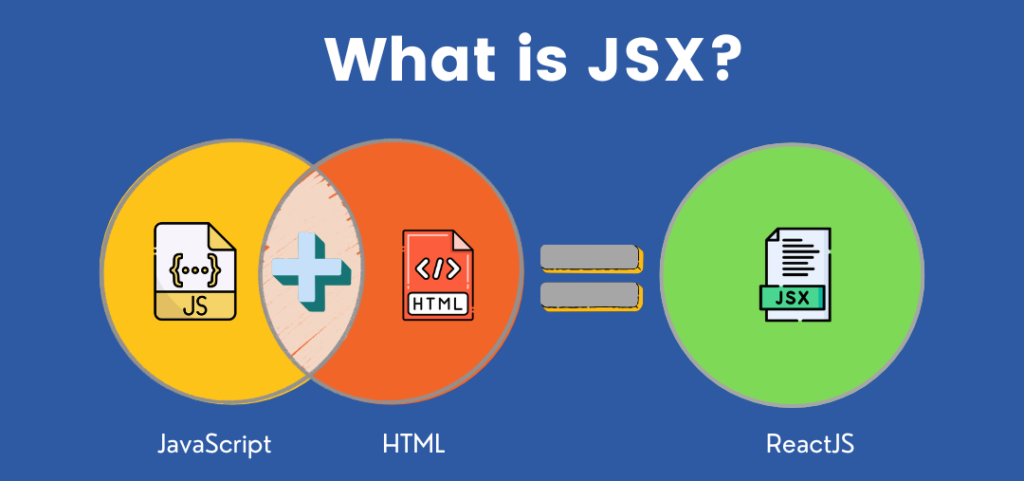
JSX stands for JavaScript XML. It allows you to write HTML-like syntax directly within JavaScript, making your code more readable and declarative. While it looks similar to HTML, JSX has the full power of JavaScript, enabling you to embed expressions and manipulate the DOM efficiently.
Writing JSX Syntax
JSX syntax is straightforward but has some differences from HTML:
- You can use JavaScript expressions within JSX by wrapping them in curly braces
{}
. - JSX tags must be properly closed, either with a closing tag (
</>
), or self-closing for elements with no children (< />
).
Here’s an example of JSX syntax:
- You can use JavaScript expressions within JSX by wrapping them in curly braces
const element = Hello, world!
;
This is how you would render this element using React:
import React from 'react';
import ReactDOM from 'react-dom';
const element = Hello, world!
;
ReactDOM.render(element, document.getElementById('root'));
Embedding Expressions in JSX
One of the powerful features of JSX is the ability to embed JavaScript expressions directly within your markup. This is done by wrapping the expressions in curly braces {}
.
For example:
const name = 'Niraj';
const element = Hello, {name}!
;
This will render as:
Hello, Niraj!
You can also embed more complex expressions:
const user = {
firstName: 'Niraj',
lastName: 'Kumar'
};
const element = Hello, {user.firstName} {user.lastName}!
;
This allows you to dynamically render content based on your application’s state or props.
JSX Attributes and Children
JSX supports attributes similar to HTML. These attributes can be used to pass data to components, just like props in React.
For example:
const element =
;
Notice the use of camelCase for attributes, like className
instead of class
and onClick
instead of onclick
.
Children in JSX
JSX allows you to nest elements inside other elements, enabling you to build complex structures.
For example:
const element = (
Hello, world!
Welcome to learning React.
);
This nests a <h1>
and a <p>
element inside a <div>
, creating a parent-child relationship.
JSX can also contain JavaScript expressions as children:
const items = ['Apple', 'Banana', 'Cherry'];
const element = (
{items.map((item) => (
- {item}
))}
);
Here, the map
function iterates over an array of items and returns a list of <li>
elements. Each list item is assigned a unique key
attribute, which is crucial for performance and managing dynamic lists in React.
Conclusion
JSX is a powerful and flexible syntax that blends HTML-like structure with JavaScript’s capabilities. It makes writing and understanding React components easier and more intuitive. By leveraging JSX, you can create dynamic, interactive UIs efficiently and effectively. As you continue to explore React, you’ll see how JSX plays a central role in building and managing your application’s user interface.
Next, we’ll dive into components, the building blocks of any React application, and learn how to create and use them effectively.